NLTK and Wordnet facilitate the extraction of synonyms and antonyms in Python. These tools import necessary libraries, use WordNet to find synsets of words, and extract lemma names for synonyms. Antonym extraction incorporates challenge of handling polysemous words and differentiating between types of antonyms. Context usage and part-of-speech (POS) tagging refine this process. Implementing custom functions with Wordnet can enhance the efficiency of extraction, with features like semantic similarity calculations. Python's NLTK and Wordnet's capabilities extend beyond English language, offering multilingual lexical relation handling. Unveiling their full potentials can open avenues for enhanced linguistic analysis.
Key Takeaways
- NLTK and Wordnet can be used in Python to find synonyms by extracting lemma names from Wordnet's synsets.
- To find antonyms, Python with NLTK and Wordnet handles challenges like polysemous words and differentiates between gradable and complementary antonyms.
- Custom functions can be created in Python to improve the efficiency of synonym and antonym extraction from Wordnet.
- POS (Part-Of-Speech) tagging in Python with NLTK and Wordnet provides more accurate synonyms and antonyms by understanding the grammatical role of words.
- Improving extraction accuracy is possible by using contextual analysis, advanced linguistic patterns, a broader corpus, fine-tuning POS tagging, and keeping Wordnet updated in Python.
Understanding NLTK and Wordnet
To gain a comprehensive understanding of how to extract synonyms and antonyms using NLTK and Wordnet, it is crucial to first grasp the fundamental concepts and functionalities of these two powerful linguistic resources. NLTK, or Natural Language Toolkit, is an invaluable resource for computational linguistics, providing tools for language processing tasks. Wordnet, meanwhile, is a large lexical database of English, invaluable for Wordnet integration for language translation. Leveraging these tools, you can achieve contextual synonym and antonym extraction. This involves mining synonyms and antonyms based on the context, thereby enabling a more accurate and nuanced understanding of language, and enhancing the effectiveness of natural language processing tasks. Understanding these resources is the first step towards mastering synonym and antonym extraction.
Synonyms Extraction With Python

How can we extract synonyms of a word efficiently using Python and NLTK's WordNet? NLTK's WordNet makes this task simpler for us. We can use different synonym search methods and synonym comparison techniques to achieve this. Here is a simple process:
- Import the necessary libraries: `from nltk.corpus import wordnet`
- Find the synsets of the word: `synsets = wordnet.synsets('word')`
- Extract the lemma names: `synonyms = [lemma.name() for syn in synsets for lemma in syn.lemmas()]`
- Eliminate duplicates: `synonyms = set(synonyms)`
This approach is efficient and straightforward, providing a list of synonyms for a given word. WordNet also allows us to find semantic relationships between words, enhancing the synonym extraction process.
Antonyms Extraction With Python
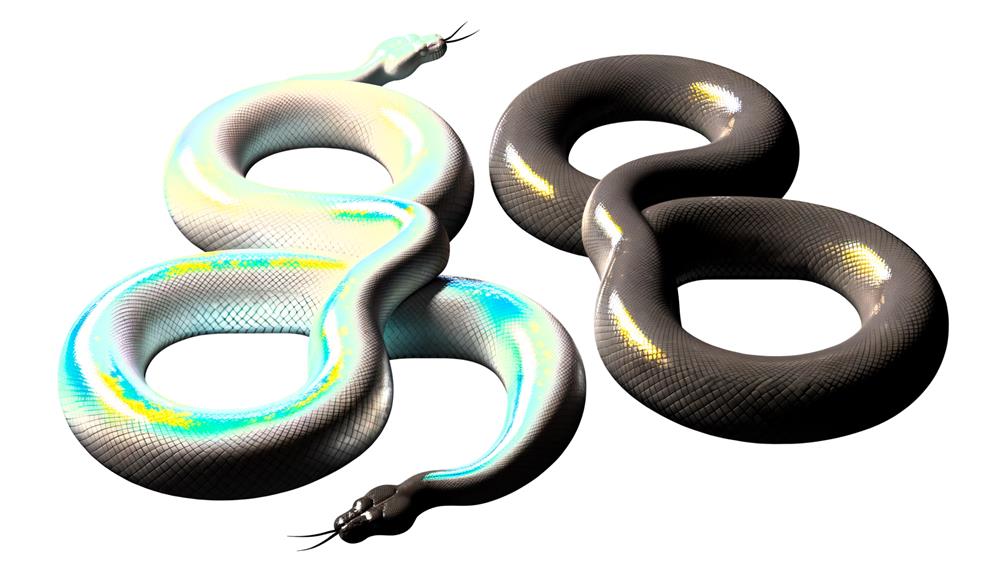
While extracting synonyms provides valuable linguistic insights, equally informative is the ability to retrieve antonyms, a task made straightforward with Python and NLTK's WordNet. By leveraging Wordnet antonyms detection techniques, Python programmers can efficiently identify opposing word pairs. However, antonyms extraction poses challenges such as dealing with polysemous words or differentiating between gradable and complementary antonyms. Innovative solutions include leveraging the context of the word usage or utilizing part-of-speech tagging to refine the extraction process. More advanced techniques might involve using machine learning algorithms to enhance the accuracy of the antonyms extraction. Thus, despite the challenges, Python and WordNet offer a powerful toolkit for antonyms extraction, furthering linguistic analysis and natural language processing tasks.
Custom Functions for Wordnet

In the realm of linguistics and natural language processing, the creation of custom functions for WordNet can significantly enhance the efficiency and accuracy of synonym and antonym extraction tasks. This can be achieved by focusing on two key areas: synonym classification and Wordnet expansion.
- Synonym classification: By creating custom functions, one can better categorize and differentiate synonyms based on their semantics and usage.
- Wordnet expansion: Custom functions can be used to extend WordNet's capabilities, allowing more comprehensive and precise retrieval of synonyms and antonyms.
- Semantic similarity: Custom functions can help in calculating the semantic similarity between words, facilitating more accurate results.
- Lexical relations: Understanding and mapping lexical relations becomes easier with custom functions.
- Efficiency: Custom functions speed up the process of finding synonyms and antonyms, making language processing tasks more efficient.
These advancements open up new possibilities for more innovative and detailed linguistics research.
POS Tagging for Synonyms and Antonyms
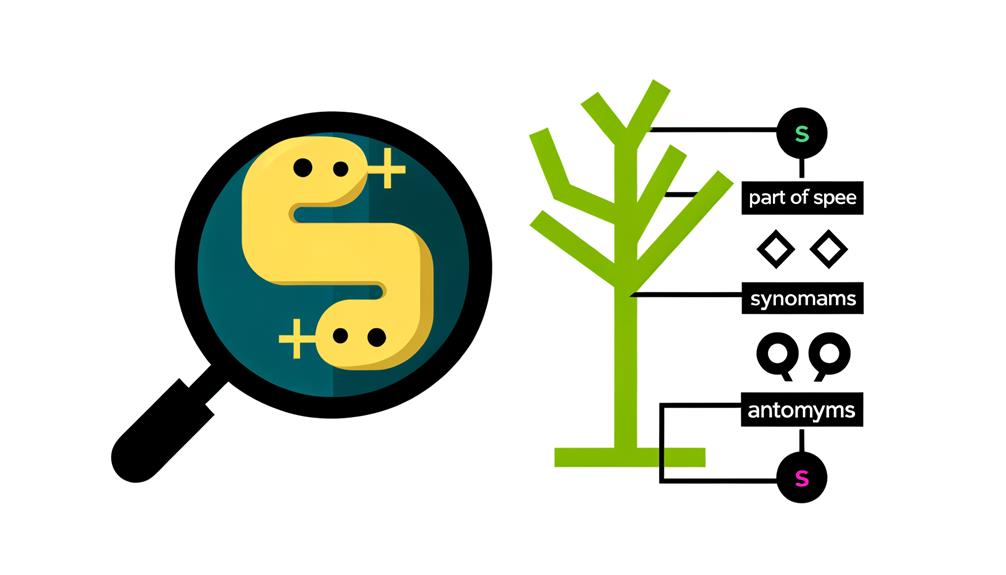
Building upon the utility of custom functions in WordNet, another important aspect to consider is the role of Part-of-Speech (POS) tagging in the extraction of synonyms and antonyms. POS tagging for synonym accuracy and enhancing antonym extraction efficiency are key ways this method is utilized. POS tags provide granular information about the grammatical role of a word in its context. This can influence the extraction process, ensuring only relevant synonyms or antonyms are retrieved. For instance, if a word is tagged as a noun, the function can be adjusted to only return synonyms or antonyms that are also nouns. This innovative approach offers a pathway towards refining the precision of semantic analysis using NLTK and WordNet, yielding results that are more contextually accurate.
Improving Extraction Accuracy
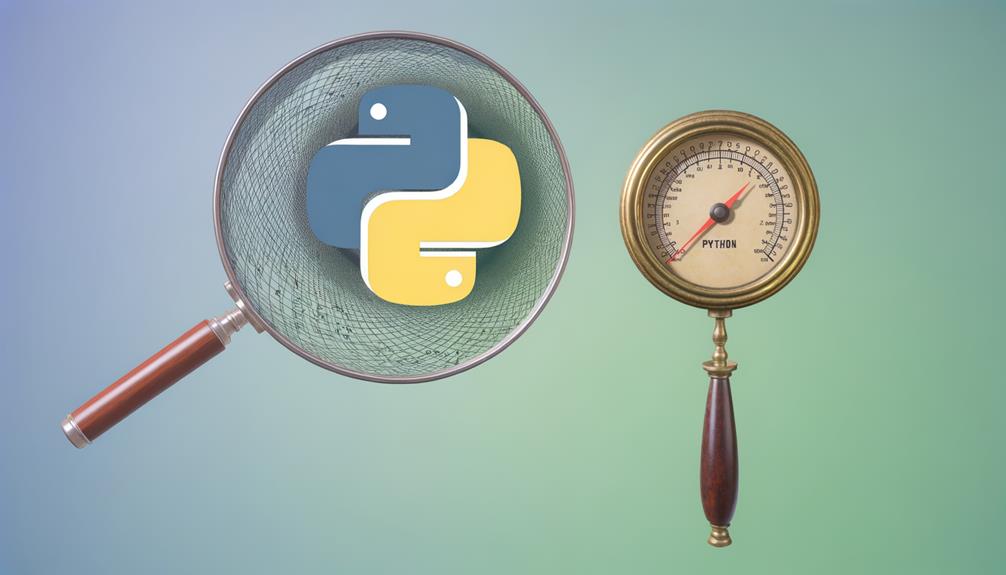
To further enhance the precision of our synonym and antonym extraction process, it's essential to consider how we can improve the accuracy of our results, particularly by refining our use of NLTK's WordNet and POS tagging functionalities.
- Contextual analysis for synonyms can avoid inappropriate matches by examining the semantic context in which words are used.
- Advanced linguistic patterns for antonyms can help identify opposites even when they aren't directly linked in WordNet.
- Using a broader corpus can increase the diversity of linguistic patterns available for analysis.
- Fine-tuning POS tagging can improve the precision of word sense disambiguation.
- Regular updates and reviews of the WordNet database can ensure our synonym and antonym extraction is grounded on the most up-to-date linguistic knowledge.
Multilingual Synonyms and Antonyms

While NLTK's WordNet is predominantly used for English language processing, it also offers an impressive array of resources for handling multiple languages, thereby providing a robust platform for extracting synonyms and antonyms in languages other than English. This feature opens avenues for translation of synonyms and antonyms across various linguistic frameworks. The integration of non-English synonym databases into NLTK's WordNet has been a major stride towards multilinguality. However, challenges arise with languages that exhibit complex linguistic phenomena, such as polysemy, homonymy, and synonymy. Despite these hurdles, NLTK's WordNet has shown versatility in handling multilingual lexical relations, thereby broadening its application spectrum beyond English language processing.
Language-Specific WordNet Resources

Despite the inherent complexities of different languages, NLTK's WordNet provides a plethora of resources specific to various languages, thereby facilitating precise and innovative extraction of synonyms and antonyms in those languages. This means that you can efficiently perform cross language synonym mapping and non-English antonym retrieval.
The following are some of the key features of language-specific WordNet resources:
- They enable multilingual synonym and antonym extraction.
- They allow for precise cross language synonym mapping.
- They facilitate non-English antonym retrieval.
- They provide language-specific synonyms and antonyms for words.
- They incorporate language nuances for more precise synonym and antonym extraction.
This opens up a world of possibilities for multilingual natural language processing tasks.
Frequently Asked Questions
What Is the Computational Complexity of Synonym and Antonym Extraction in NLTK Wordnet?
The computational complexity of synonym and antonym extraction in NLTK WordNet largely depends on the Wordnet algorithms used. It's commonly viewed as linear, or O(n), with 'n' being the total number of synsets or lemmas. However, complexity can increase with the depth of the lexical relations. Considering the intricacies of computational language processing, further empirical analysis could provide a more comprehensive understanding of the exact computational complexity involved.
Can NLTK Wordnet Handle Slang or Regional Dialects for Synonym and Antonym Extraction?
NLTK Wordnet primarily focuses on formal and standard language, which poses slang interpretation challenges. It does not inherently handle slang or regional dialects for synonym and antonym extraction. However, with advanced dialect recognition techniques and additional linguistic resources, it may be possible to extend its capabilities. This would require innovative solutions to accurately process and interpret non-standard language forms.
How Does NLTK Wordnet Handle Homonyms and Homographs in Synonym and Antonym Extraction?
NLTK WordNet employs intricate linguistic strategies to manage homonyms and homographs in synonym and antonym extraction. The impact of homonyms on NLTK is mitigated by contextual analysis, while homographs in WordNet are handled through sense disambiguation techniques. This ensures accurate extraction and interpretation of words with multiple meanings or identical spellings, providing a robust tool for linguistic analysis and natural language processing tasks.
How to Use NLTK Wordnet for Semantic Similarity Measurement Between Words?
NLTK Wordnet can be utilized for semantic similarity measurement between words, an essential task in Natural Language Processing. This involves comparing the semantic meaning of words based on their position in a lexical database. Semantic similarity applications include Sentiment Analysis, where the sentiment expressed in text is determined. Utilizing NLTK Wordnet in Sentiment Analysis can enhance the accuracy of detecting positive, negative, or neutral sentiments.
What Are the Limitations or Challenges When Extracting Synonyms and Antonyms With NLTK Wordnet?
Extracting synonyms and antonyms with NLTK Wordnet can be a complex task. A discernible limitation lies in Wordnet's accuracy, as it may not always provide contextually accurate synonyms or antonyms. Furthermore, NLTK Wordnet's scalability can pose a challenge, especially when dealing with large volumes of data. It requires significant computational resources for efficient processing, potentially limiting its usage in expansive, data-intensive applications.