To translate a website within a pandas dataframe using Python, you'll want to employ the google_trans_new package. Start by importing it and creating a Google translator object. As you navigate through your pandas dataframe, use the 'translate()' function on each entry, letting the package's automatic language detection capabilities handle a lot of the heavy lifting for you. To streamline the process, consider using the 'apply()' function with a lambda for translations, or even 'applymap' function for a full dataframe translation. Take note, it's a journey. There's always more to learn as you delve into this task.
Key Takeaways
- Import 'google_trans_new' and 'pandas' modules in Python to enable language translation and data manipulation.
- Create a translator object using 'google_translator()' from 'google_trans_new' for translation capabilities.
- Use 'apply()' function on the dataframe column that contains the website content, implementing a lambda function for translation.
- Leverage the 'translate()' method from the translator object on each row in the dataframe, specifying source and target languages.
- For automatic language detection, use 'langdetect' module, which can identify over 55 languages, and combine it with the 'translate()' function.
Understanding Python Language Translation
Let's dive into how you can leverage the google_trans_new Python package to translate a sentence from one language to another, thereby unlocking a world of multilingual data processing possibilities. This Python package is a powerful tool that eases language detection and translation. To get started, you first import the 'google_translator' function from the package. You then create a translator object by assigning 'google_translator()' to a variable. With your translator ready, you use the 'translate()' method, specifying the source and target languages with 'lang_src' and 'lang_tgt' respectively. If you leave 'lang_src' blank, the python package automatically detects the language, offering you seamless, efficient translation across a multitude of languages.
Translating a Single Sentence
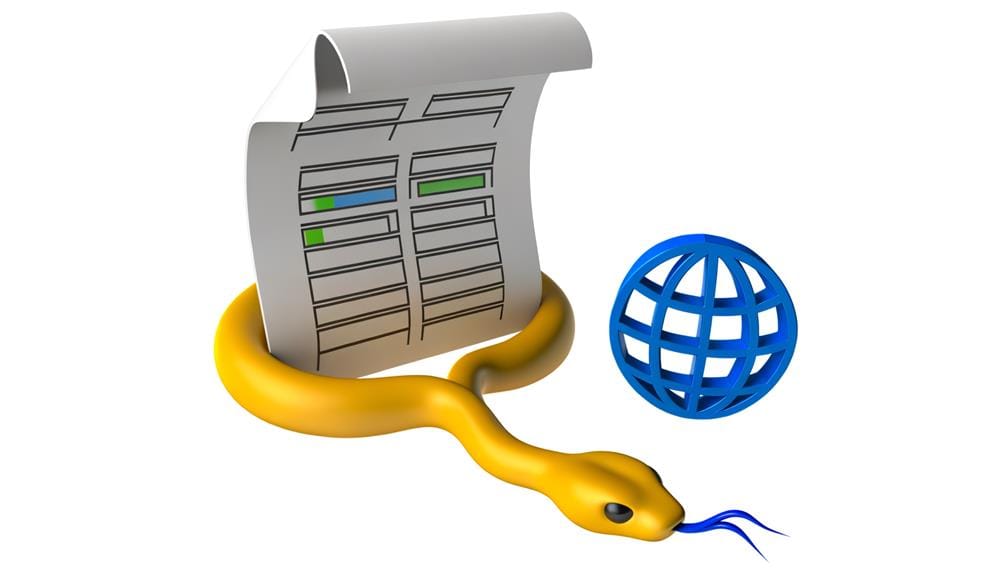
To begin translating a single sentence, you first need to select the text you wish to translate. Then, leverage the 'google_translator' function and 'translate()' method, setting your source and target languages accordingly. This process simplifies translating phrases and utilizes built-in language detection techniques.
Here are the steps to follow:
- Import the 'google_trans_new' module and instantiate the 'google_translator' function.
- Choose the sentence you'd like to translate and assign it to a variable.
- Use the 'translate()' method on your chosen sentence.
- Set 'lang_src' to the original language and 'lang_tgt' to the desired language.
- Lastly, print the result to view your translated text.
Translating a Dataframe Column
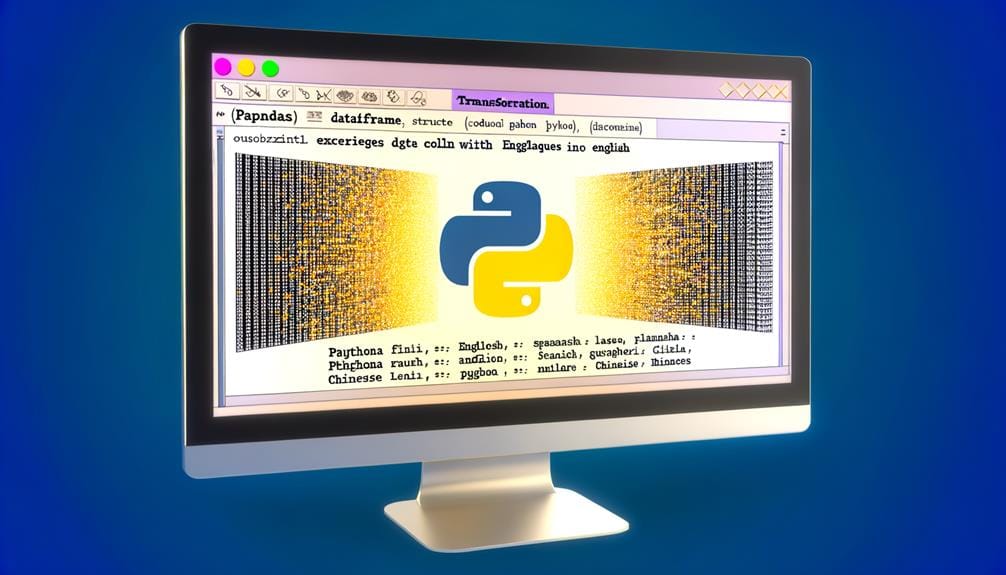
Diving deeper into Python's powerful capabilities, you can leverage the google_trans_new package to translate an entire column in a pandas dataframe, streamlining the process for large datasets. This innovative approach significantly reduces manual effort while improving translation accuracy.
Firstly, you'll need to instantiate the Google Translator. Then, select the specific column to translate by applying the translator function to each row. It's essential to carefully consider your language selection strategies, as accurate language detection is crucial for the success of translation.
This technique is flexible and scalable, accommodating large datasets with ease. By translating a dataframe column, you are making your data more accessible and inclusive, ultimately enabling more comprehensive data analysis.
Translation Without a for Loop
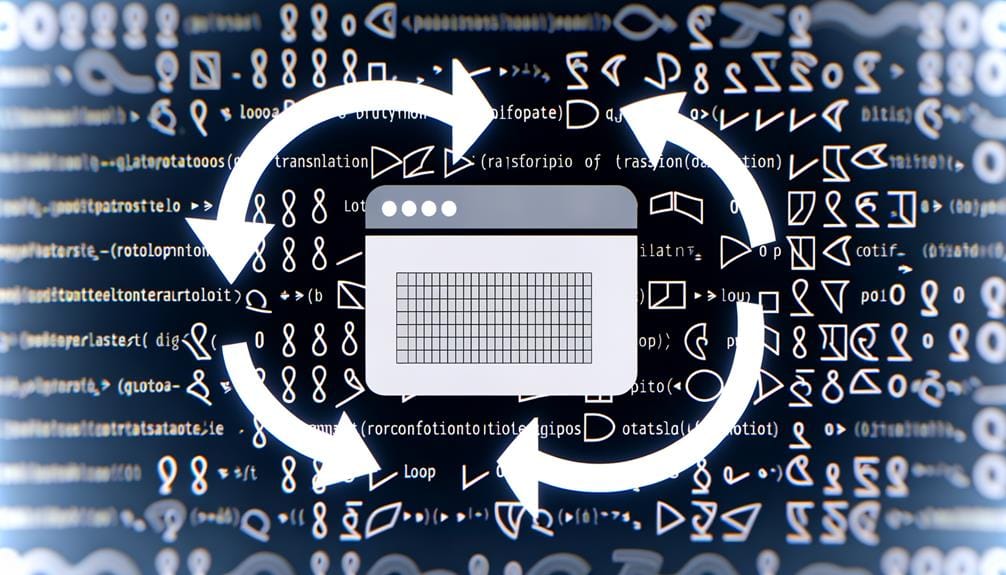
Imagine effortlessly translating an entire column of a dataframe without resorting to a for loop, a feat made possible by harnessing the power of Python's apply method combined with a lambda function. This technique ensures efficient translation and proper language detection, making your code run faster and cleaner.
Here's what you need to know:
- Use the `apply()` function on the dataframe column you intend to translate.
- Implement a lambda function to pass each cell to the translator function.
- Ensure that the translator function can handle any data type.
- Handle possible exceptions to maintain the flow of translation.
- Resulting translations are directly stored in the dataframe, eliminating the need for additional storage variables.
Embrace this innovative, detail-oriented, and analytical approach to make your translations more efficient.
Full Dataframe Translation Method
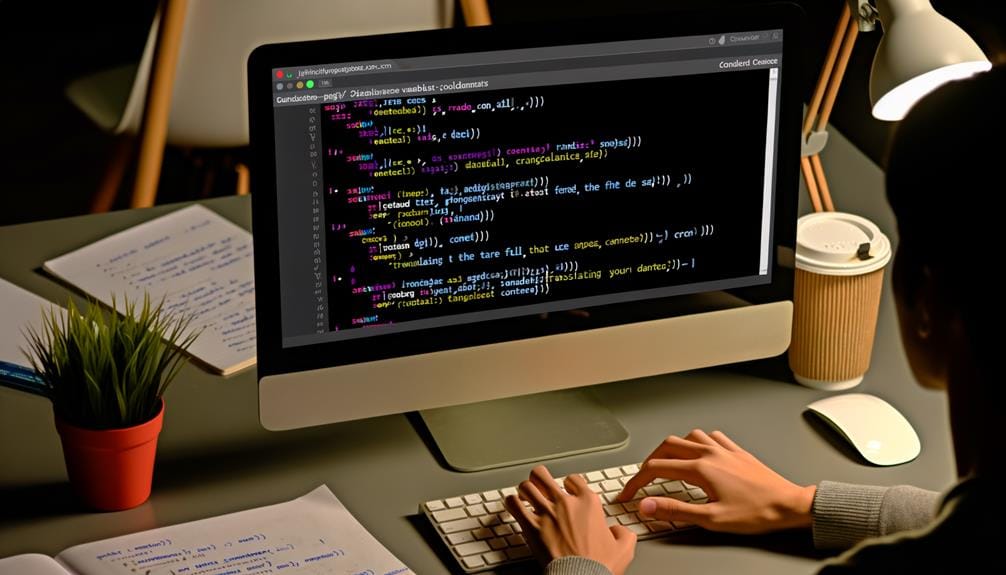
Building on the efficiency of translating individual columns, you can also apply Python's powerful capabilities to translate an entire dataframe, offering a comprehensive solution for your multilingual data processing needs. This approach is central to effective website translation strategies, and it significantly enhances multilingual website optimization.
Consider this simple dataframe, for example:
Column 1 | Column 2 |
---|---|
Hello | World |
Goodbye | Earth |
Welcome | Universe |
Farewell | Galaxy |
Hi | Planet |
In Python, you could use the 'applymap' function to translate every cell in the dataframe. This function applies a function to every single element in the dataframe, making it ideal for comprehensive translations. However, be mindful of the API usage limit to avoid potential issues.
Automatic Language Detection
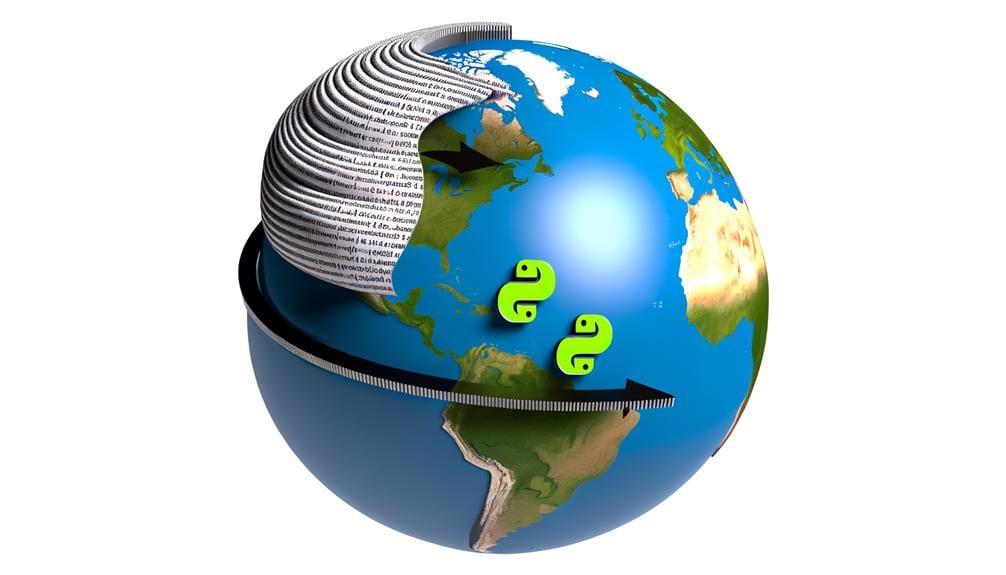
In the exciting world of language translation with Python, you'll find that automatic language detection is an incredibly innovative and crucial feature. It's a game-changer for website translation, enabling you to identify the language of a text without any prior information.
Here are some key aspects to consider:
- The `langdetect` module offers reliable language identification.
- It employs Google's language-detection library, which supports over 55 languages.
- You simply input your text, and it outputs the probable language.
- When dealing with multiple languages, it can identify each one separately.
- It can be combined with the `translate()` function for seamless translation.
This feature adds an extra dimension to your Python-based translation toolbox, ensuring more comprehensive and accurate translations.
Website Content to Dataframe Conversion
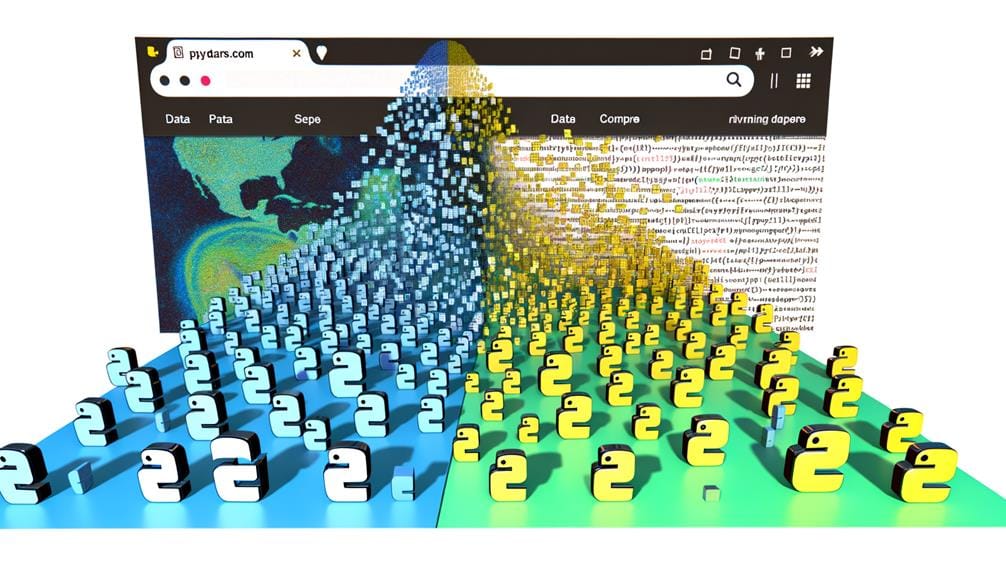
To convert a website's content into a dataframe for translation, you'll first need to employ an SEO crawler such as OnCrawl, JetOctopus, Ahrefs, or Screaming Frog, which can effectively capture the entirety of a site's textual data. This process, known as website content extraction, is fundamental in translation projects.
Next, you'll need to utilize language detection techniques to identify the original language of the website content. It's crucial for the translation process as it ensures accuracy.
Here's a sample data representation:
URL | Original Language | Content |
---|---|---|
www.example1.com | English | "Hello World" |
www.example2.com | Spanish | "Hola Mundo" |
www.example3.com | French | "Bonjour le monde" |
www.example4.com | German | "Hallo Welt" |
Effective Website Crawling for Translation
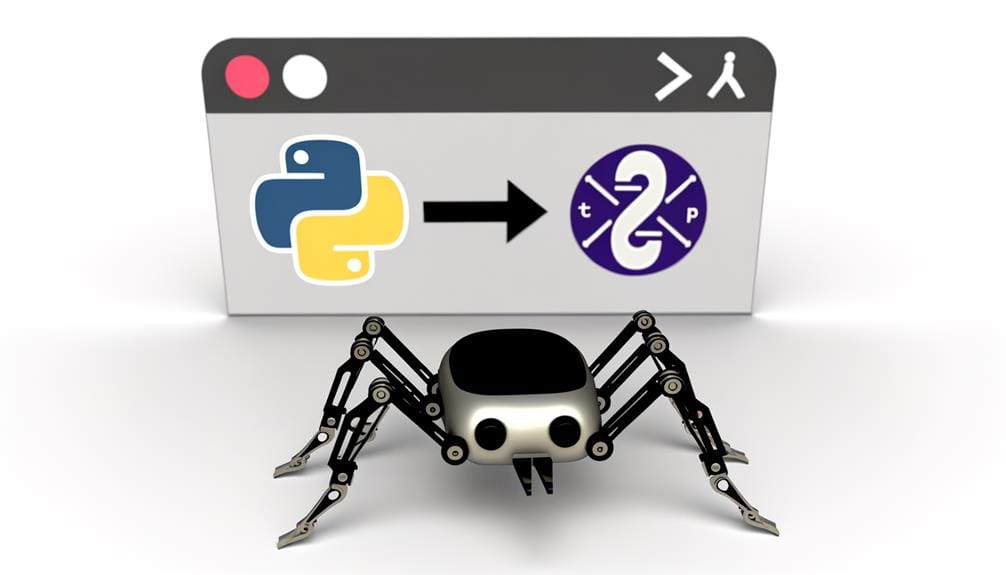
Often, the success of a translation project hinges on your ability to effectively crawl the website, capturing all textual data accurately and thoroughly for the subsequent translation process. Here are some techniques that can help you achieve this:
- Utilize SEO Crawlers: Tools like OnCrawl, JetOctopus, Ahrefs, or Screaming Frog can be effective in crawling the website.
- Use Advertools' crawl() function: This can capture a website's content entirely.
- Consider semantic similarity: This enhances the effectiveness of your translation.
- Consider the content type: Different types of content may require different translation approaches.
- Follow guidelines: Guidelines for website crawling and SEO analysis can be invaluable.
Frequently Asked Questions
What Are the Limitations of Using Google_Trans_New for Language Translation?
While google_trans_new offers handy translation tools, it's not flawless. You may find issues with translation accuracy, especially with complex sentences or idioms. Also, there're API restrictions to consider. Google can limit or block your IP if you're making too many requests in a short time period. Always be innovative in combining multiple translation APIs or methods to overcome these limitations and increase the efficiency of your Python translation tasks.
How to Handle Errors During the Translation of a Dataframe Column?
When handling errors during dataframe column translation, you'll want to incorporate error debugging and exception handling techniques. Start by identifying the error type: is it a syntax error or an exception? Then, use try/except blocks in your code to catch and handle these exceptions. Be innovative: instead of stopping the translation process, you can log the error and continue with the next row. This approach ensures your program remains robust and efficient.
Can Python Perform Real-Time Translation of Website Content?
Yes, Python can perform real-time translation of website content. Its efficiency and the accuracy of translation modules, such as google_trans_new, make this possible. You'd first need to convert the website content into a pandas dataframe. Then, Python can be used to translate the entire dataframe or specific columns into the desired language. It's a detailed-oriented, innovative approach that provides accurate, real-time translations.
How to Maintain the Original Format of the Text During Translation?
To maintain the original format during translation, you'll need to consider text encoding and format preservation. Python's 'html' package can help preserve HTML tags, ensuring format stays intact. For other text types, consider using 're' or 'regex' packages to identify and protect formatting elements. Always ensure your encoding matches the source content to avoid losing special characters or accents during translation. Be creative and innovative when preserving format; it's key to accurate translation.
What Are the Ethical Considerations When Crawling a Website for Translation?
When crawling a website for translation, it's crucial you respect privacy and ensure data security. Always ask for permission before accessing private data. Don't store personal information without consent. Use secure methods to handle data, protecting it from unauthorized access. Be innovative, create systems that respect user privacy while providing efficient translation services. Analyze each step to ensure you're not infringing on rights or compromising security.