We can list all the files in a directory using Python's OS module. Specifically, the os.scandir() function or os.walk() function are frequently used. Using these functions, you can access all files and folders, and even traverse subdirectories. If you want an object-oriented approach, Python's Pathlib module might be more suitable. Advanced techniques include the usage of generators, and filtering files with functions such as fnmatch.fnmatch(). Additionally, file attributes like size or modification time can be fetched with os.stat(). Stick with us to discover more about Python's powerful file handling capacities.
Key Takeaways
- You can use the os.listdir() function in Python's os module to list all files in a directory.
- For a hierarchical view of files, use os.walk() to traverse directories and subdirectories.
- Python's os.scandir() function efficiently scans and retrieves directory entries, including files and subdirectories.
- The Pathlib module provides object-oriented methods for file manipulation, including listing of files.
- To filter files in a directory, use Python's fnmatch.fnmatch() function or regex patterns.
Understanding the OS Module
To fully grasp the power of Python's file handling capabilities, we must first delve into understanding the OS module, a vital tool for interacting with the operating system. This module is a treasure trove of functionalities that allow us to perform operating system dependent tasks, exploring functionalities such as reading, writing, and modifying files and directories. It's a practical application for tasks like listing all files in a directory or renaming a file. We can determine the current working directory, change it, or even create and remove directories. By harnessing the power of the OS module, we're able to interact with the file system, manipulate paths, and handle files and directories. This understanding forms the foundation for more advanced file handling techniques in Python.
Using Os.Walk() Function
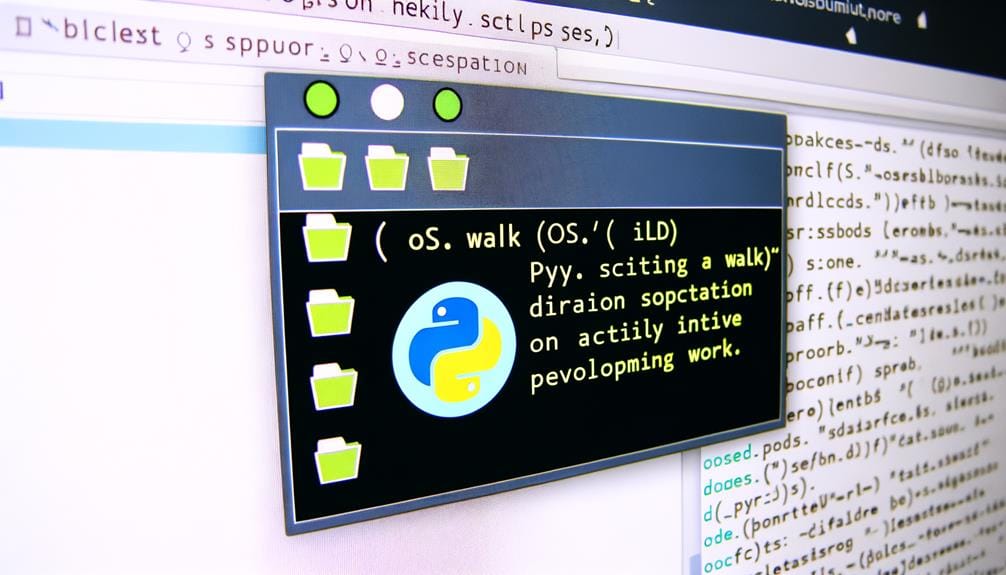
Diving into the depths of Python's capabilities, we encounter the os.walk) function, a powerful tool for traversing directories and listing files, including those nested within subdirectories. This function is a gem for recursive file listing, effortlessly reaching into every corner of our directories, no matter how deeply nested. It yields a three-tuple, producing the directory path, a list of subdirectories, and a list of files in the current directory. This return structure makes it ideal for file metadata retrieval, as it provides comprehensive data about our folders and files. It's important to note the os.walk() function doesn't automatically sort these lists, giving us flexibility to manipulate and organize the data as we see fit. This function truly exemplifies the robust and efficient nature of Python's filesystem handling capabilities.
Listing Files With Nested Loops
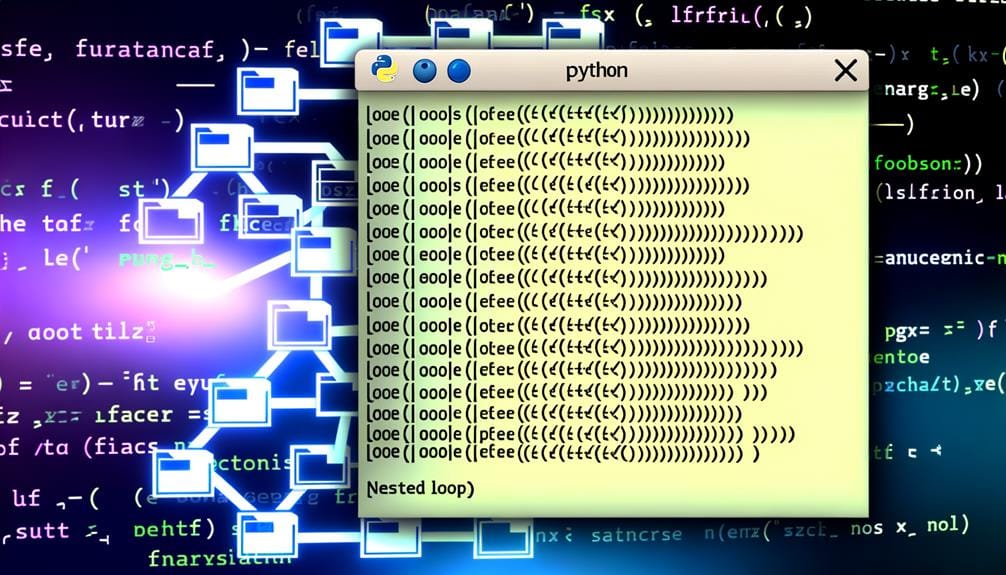
Building on our understanding of Python's file system handling, we'll now delve into the practical application of nested loops for listing files in a directory. This technique is especially useful for file organization and recursive directory listing.
Consider the following steps:
- We initiate with Python's built-in `os` module to interact with the operating system.
- We employ `os.walk()`, a handy function that generates filenames in a directory tree.
- Inside the main loop, we construct a nested loop to access each file in the directory and its subdirectories.
- Finally, we print out the file paths.
Using nested loops, we can efficiently traverse and list all files, even in complex directory structures. This allows for a comprehensive, organized view of our files, making our coding life easier.
Role of Generators in File Listing
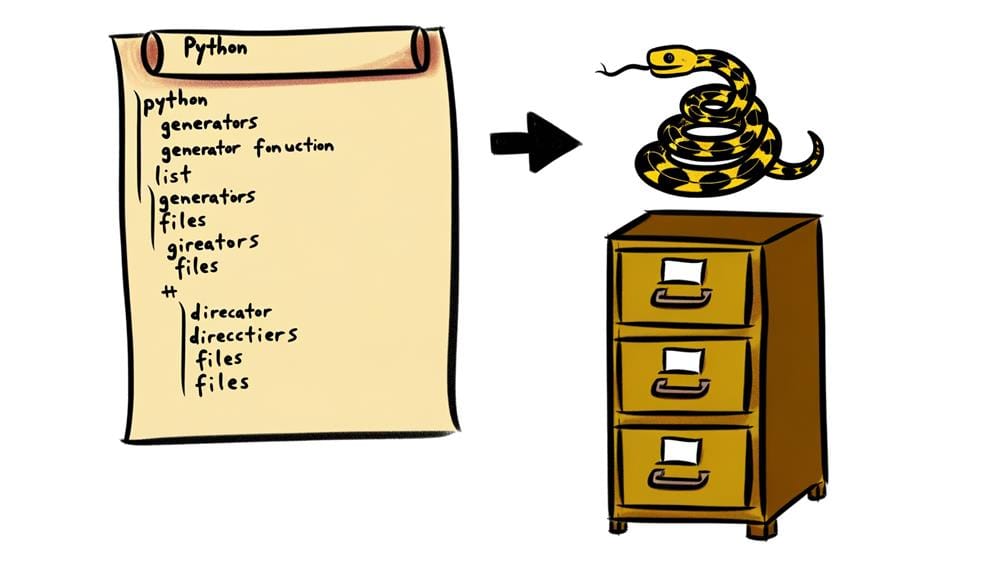
Let's now explore the role of generators in Python, which can significantly streamline the process of listing files in a directory. Generators, due to their on-demand computation ability, help overcome file listing challenges such as memory limitations. They allow us to handle large directories efficiently, improving performance.
Here's a comparison of generator applications and traditional methods:
Method | Performance | Memory Usage |
---|---|---|
Generators | High | Low |
Traditional Loops | Low | High |
List Comprehensions | Medium | High |
Generators provide solutions to file listing challenges, such as handling large datasets and improving code readability. They allow us to iterate over large directories without loading everything into memory, making our code more efficient and manageable.
Introduction to Os.Scandir() Function
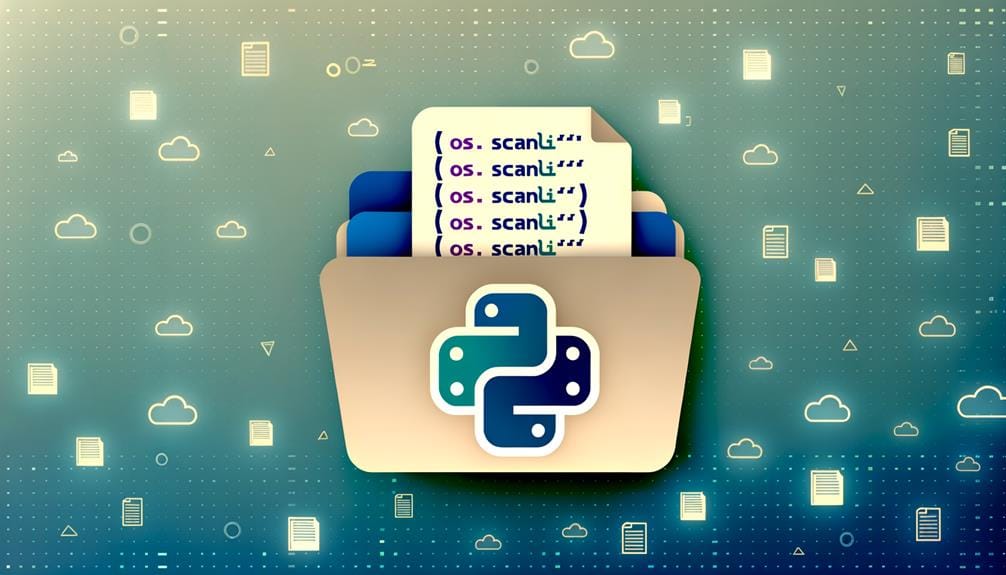
In the realm of Python, the os.scandir() function emerges as a powerful tool for scanning an entire directory or folder, including its subdirectories, thus equipping developers with a comprehensive list of all files and elements. This function is a game-changer for performance optimization as it is faster and more efficient compared to its predecessor, os.listdir().
- It provides detailed information about each file, minimizing the need for additional system calls.
- It offers effective error handling strategies, enhancing the robustness of our code.
- It yields better performance even with large directories.
- It allows easy identification of file types, contributing to more efficient filtering and sorting.
With os.scandir(), we're given a reliable, performance-optimized tool that makes navigating through directories a breeze.
Filtering Files With Fnmatch.Fnmatch()
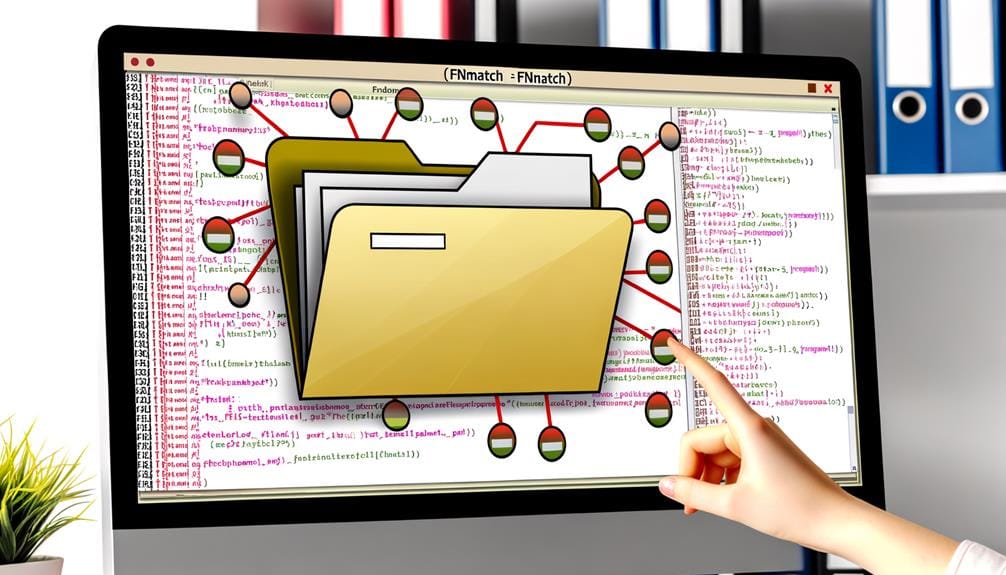
Often, we find ourselves needing to filter files based on their names or types, and that's where the fnmatch.fnmatch) function in Python becomes incredibly useful. This function provides a simple method for pattern matching, allowing us to filter through files more efficiently. With fnmatch.fnmatch(), we can match names using Unix shell-style wildcards, which makes it a powerful tool for file type filtering. For instance, to filter all '.txt' files in a directory, we'd use fnmatch.fnmatch(file, '*.txt'). This command would return either True or False, letting us know if the file matches our pattern. This way, we can sift through a sea of files, quickly identifying those that meet our specific criteria.
Utilizing Regex Patterns for Filtering

While fnmatch.fnmatch) provides a simple method for pattern matching, there are times when we need a more comprehensive solution, and this is where regex patterns come into play for filtering files. Regex, or regular expressions, provide a powerful tool for complex pattern matching and can be used to create custom filter patterns based on file names.
- Regex allows us to specify complex patterns that can match any combination of characters in a file name.
- It's highly versatile, allowing us to filter different file types with ease.
- Regex patterns can be used to create intricate filters that fnmatch.fnmatch() can't handle.
- It can also be used to filter files based on their content, not just their names.
This gives us a much greater control over our file filtering process.
Exploring Pathlib Module Functions
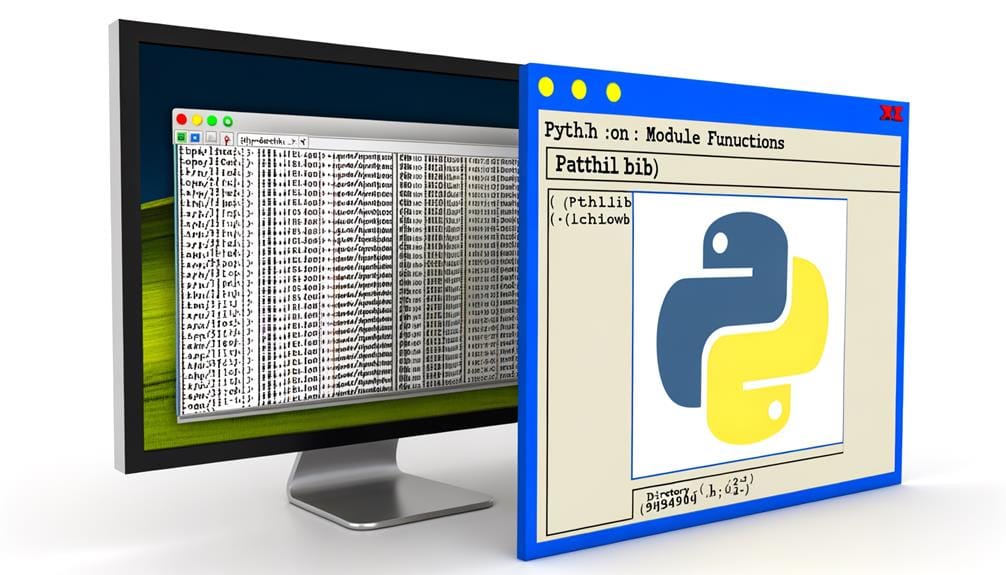
Diving into the depths of Python's Pathlib module, we discover a treasure trove of functions that streamline file and directory manipulation. The Pathlib module revolutionizes path handling with its object-oriented approach, replacing traditional string manipulation with more powerful and intuitive methods. For instance, the 'Path' class provides a flexible way to navigate the file system, with methods like 'iterdir' to loop over each item in a directory. 'Glob' method, on the other hand, enables pattern matching, making it easier to find and manipulate files of a certain type. Furthermore, 'resolve' offers a way to get absolute paths, and 'exists' checks if the path points to an existing file or directory. With Pathlib, we've got a robust tool for handling complex file manipulation tasks.
Use Cases of File Listing Techniques
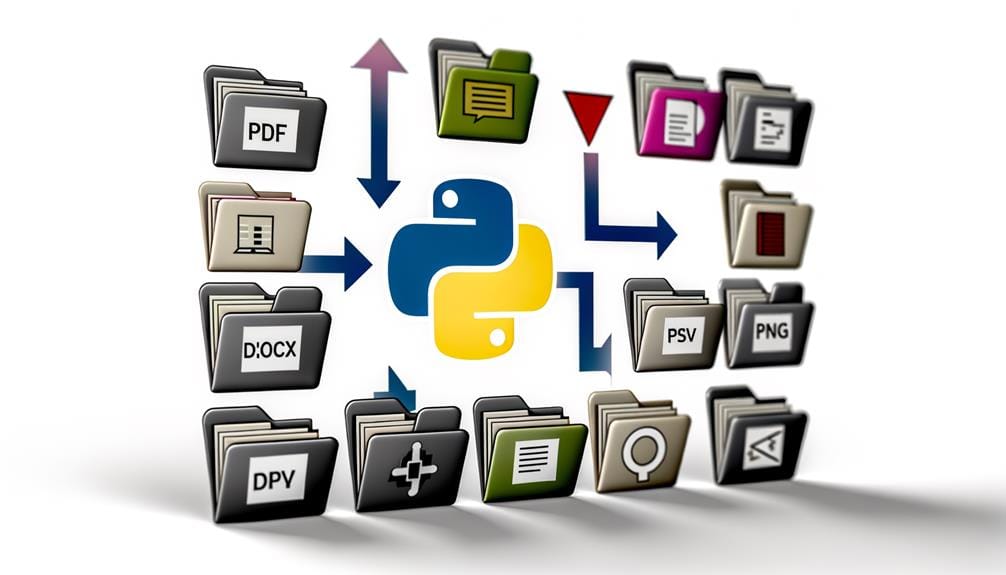
Unleashing the power of Python's file listing techniques, we find a myriad of practical uses across various domains, from organizing files in a project, to optimizing images for SEO, to handling specific file types in bulk for data science tasks. These techniques provide a framework for successful file organization and bulk file handling.
- In web development, listing techniques help organize project files, making it easier to locate and manage resources.
- SEO specialists optimize images, often handling hundreds of files at once. Python's file listing techniques streamline this process.
- Data scientists often deal with datasets stored as files. Python's techniques allow for efficient handling of these files.
- For system administrators, these techniques aid in automating tasks such as backup management or system monitoring, enhancing efficiency and productivity.
Advanced File Listing Techniques
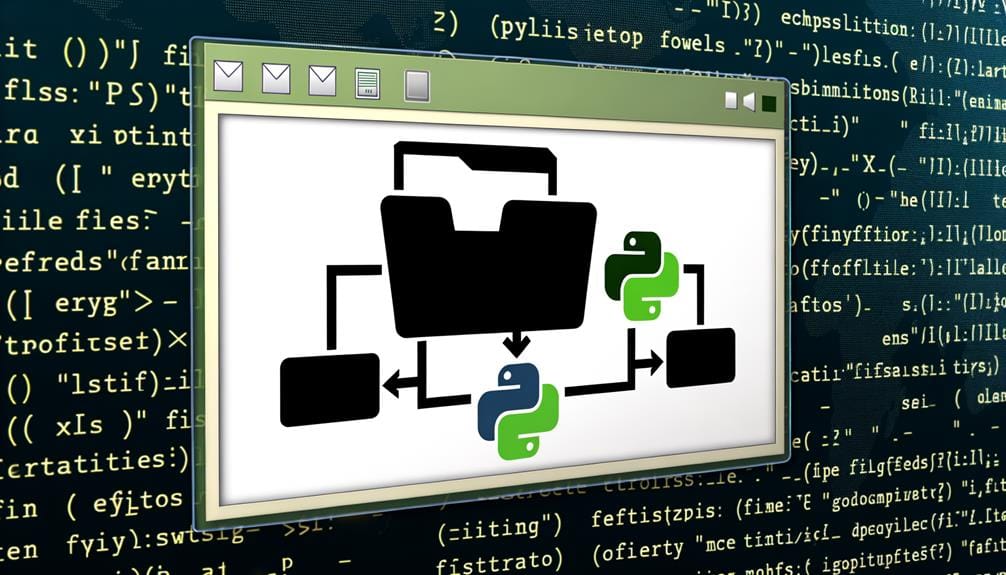
Let's explore some advanced techniques for file listing in Python, which take our file management capabilities to a whole new level by offering precision, customization, and efficiency. We can leverage recursive listing to navigate through all subdirectories, ensuring we don't miss any files hidden in nested folders. File attributes such as size, modification time, and type can be fetched using the os.stat() function, providing deeper insights about our files.
Here's a quick overview:
Technique | Description |
---|---|
Recursive Listing | Navigate through all subdirectories using os.walk(). |
File Attributes | Fetch file attributes like size, modification time, type using os.stat(). |
Fostering Engagement Through Interaction
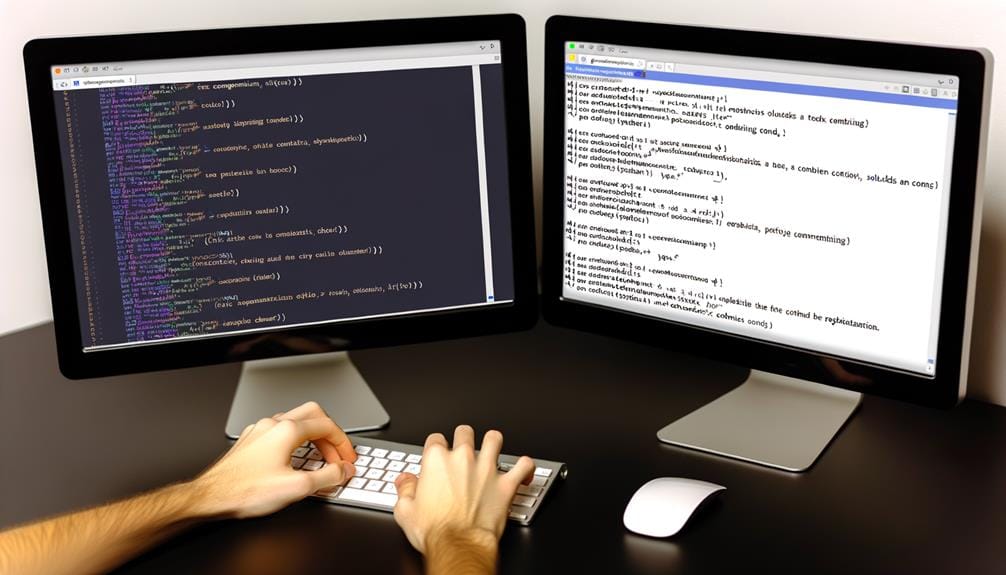
While advanced techniques enhance our file listing capabilities in Python, fostering a sense of community and engagement through interaction can further enrich our learning experience in this technological domain. We believe in the power of community engagement and understand that our readers' feedback is invaluable.
- Facilitating open forums for discussion to foster active engagement.
- Encouraging readers to share their unique insights and experiences.
- Valuing reader feedback to enhance content quality and relevance.
- Promoting interactive learning through shared problem-solving.
Frequently Asked Questions
What Are the Differences Between Os.Walk() and Os.Scandir() Functions in Python?
We've noticed that os.walk() and os.scandir() in Python differ in performance. In speed tests, os.scandir outperforms os.walk, especially in large directories. For recursive traversals, os.walk is more suitable, while os.scandir excels in shallow scans. When deciding between os.walk and os.scandir, the choice hinges on the specific scenario and efficiency required.
How Can I Handle Errors During the File Listing Process in Python?
We're often faced with errors during the file listing process in Python. To handle these, we'll use Error Handling Basics and Python Exception Handling. We wrap our code in a try/except block, allowing us to catch and respond to any errors that may occur. This makes our code more robust, ensuring continuity even when unexpected issues arise. In our experience, it's an essential practice in any Python project.
Can I Use These File Listing Techniques With Cloud Storage Like Google Drive or Dropbox?
Yes, we can use these file listing techniques with cloud storage like Google Drive or Dropbox. We'd need to interact with their cloud storage APIs and handle authentication. After we're authenticated, we can list, upload, or download files. It's not as straightforward as local file handling, but it's definitely doable. Keep in mind, each cloud storage service has its own API, so the specific implementation might vary.
How Can I List and Filter Files Based on Their Modification or Creation Date?
We can certainly list and filter files based on their modification or creation date. It's all about using the right metadata extraction techniques. Python's os.path module provides methods like getmtime and getctime that fetch modification and creation times respectively. Coupled with filtering file extensions, we can create an efficient, detailed analysis of our directory, yielding precise, useful results. It's an insightful way to manage and understand our files.
Is There a Way to List Files From Multiple Directories Simultaneously in Python?
Yes, we can list files from multiple directories simultaneously in Python. We'll use the glob library for this task. It provides a function, glob.glob), which supports recursive file listing. By passing "**" as part of the path and setting the recursive parameter to True, we're able to retrieve files from all subdirectories. It's a powerful tool for handling multiple directories at once.