We're using Python libraries like Pillow and OpenCV for efficient image resizing. They help optimize images, improving your website's performance and SEO rankings. Additionally, writing a script for bulk image resizing can automate the process, while dynamic parameters maintain image quality. Our guidelines even tackle challenges around handling large images by using parallel processing techniques. As you progress through our tutorial, you'll understand how image resolution impacts user experience and discover ways to optimize resizing scripts further. There's more in-depth knowledge awaiting, so keep going!
Key Takeaways
- Python libraries like Pillow, OpenCV, and scikit-image are essential for image resizing tasks.
- Bulk image resizing improves website optimization, enhancing user experience and SEO rankings.
- Resizing parameters are implemented in scripts to maintain image quality and consistency.
- Optimizing resizing scripts through dynamic parameter specification and error handling enhances performance and flexibility.
- Parallel processing can significantly reduce time taken for resizing large images, optimizing CPU and memory usage.
Python Libraries for Image Resizing
Diving into the realm of image resizing, it's essential to understand the powerful Python libraries available for this task, each offering unique features and functionalities tailored for various image manipulation needs. Let's first focus on Pillow and OpenCV, two predominant libraries. Pillow provides handy image compression techniques, while OpenCV excels in maintaining aspect ratio preservation during resizing. These libraries allow us to manipulate image size without compromising quality, a crucial factor in digital media. They also support a wide range of image formats, which adds to their versatility. It's important to note that while both libraries can perform similar tasks, each has its own strengths. So, understanding these libraries and their capabilities is a significant first step in mastering image resizing.
Understanding Bulk Image Resizing
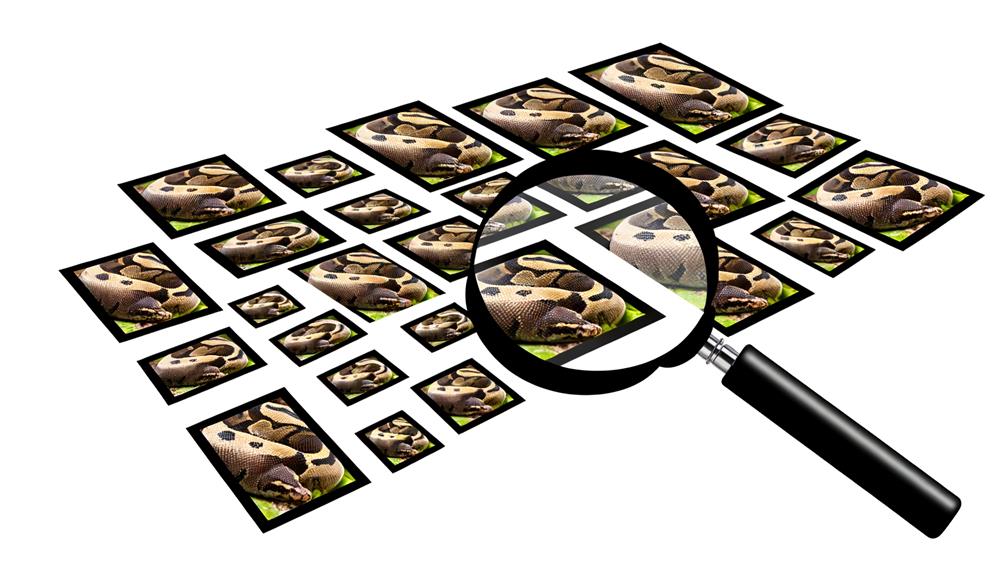
Shifting our focus to bulk image resizing, it's crucial to grasp how this method improves website optimization, enhances user experience, and saves significant storage space. We'll delve into this by discussing three main points:
- Image compression techniques: Bulk image resizing leverages various techniques to compress images while maintaining their quality. This reduces load times, boosting site performance.
- Consistency in image resolution: Bulk resizing ensures uniformity in image resolution across your site, leading to a consistent user experience.
- Storage space efficiency: By reducing image sizes, we minimize the storage space consumed, which is beneficial, especially when dealing with a large number of images.
Understanding the impact of image resolution on website performance and mastering image compression techniques are integral aspects of bulk image resizing.
Importance of Efficient Image Resizing
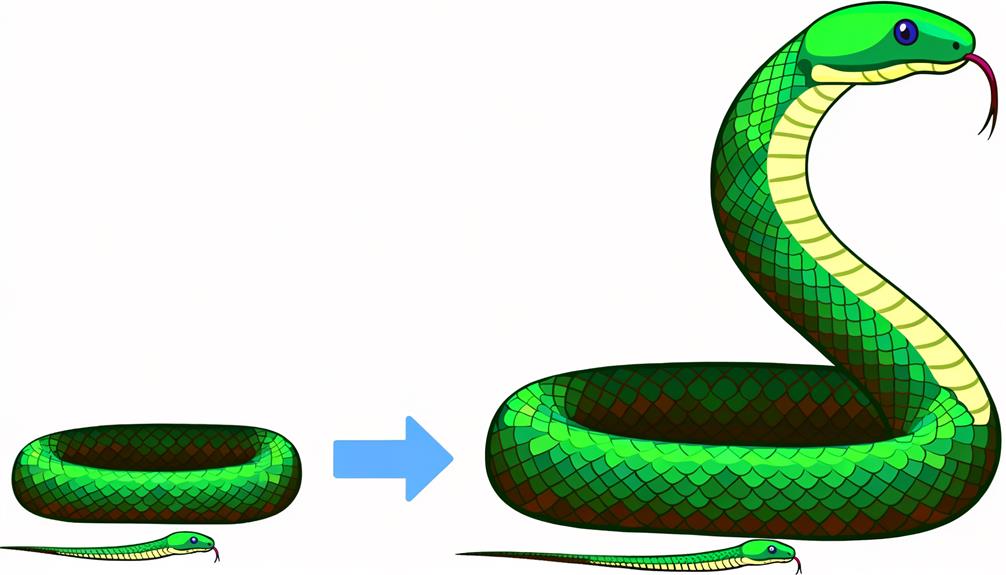
In the realm of digital imaging, efficient image resizing plays a pivotal role, not only in optimizing web performance but also in enhancing visual consistency and saving crucial storage space. Image compression techniques are integral to this process as they reduce file size without significantly compromising the quality of the image. This helps to speed up website load times, improving user experience and SEO rankings. Efficient resizing also plays a vital role in achieving mobile responsiveness. As we're living in an age where more people access the internet through their smartphones than desktops, ensuring images scale well to different screen sizes is essential. It's clear that mastering efficient image resizing is a valuable skill in the modern digital landscape.
Installing Python Libraries for Resizing
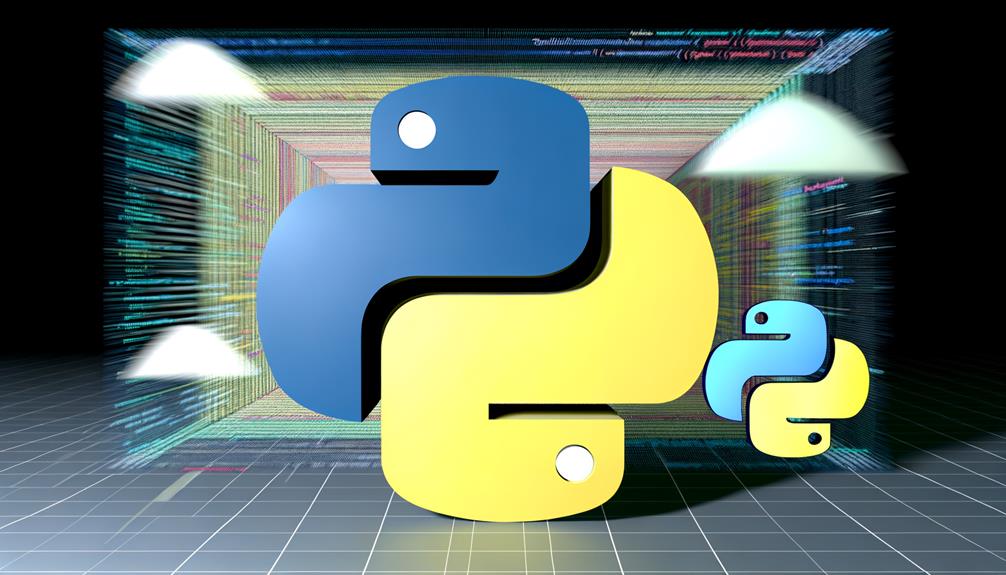
After understanding the importance of efficient image resizing, we can now start setting up our Python environment for the task by installing the necessary libraries. The Python library installation is a straightforward process and is a crucial step in implementing image resizing techniques.
To get started, here are three essential libraries we'll need:
- `Pillow`: This is an advanced fork of PIL (Python Imaging Library). It supports opening, manipulating, and saving many different image file formats.
- `OpenCV`: OpenCV (Open Source Computer Vision Library) is used for machine learning and image processing. It's highly efficient and has powerful tools for image resizing.
- `scikit-image`: This library is great for image processing in Python. It's easy to use and works well with NumPy arrays.
These libraries will lay the groundwork for our image resizing tasks.
Writing a Bulk Image Resizing Script
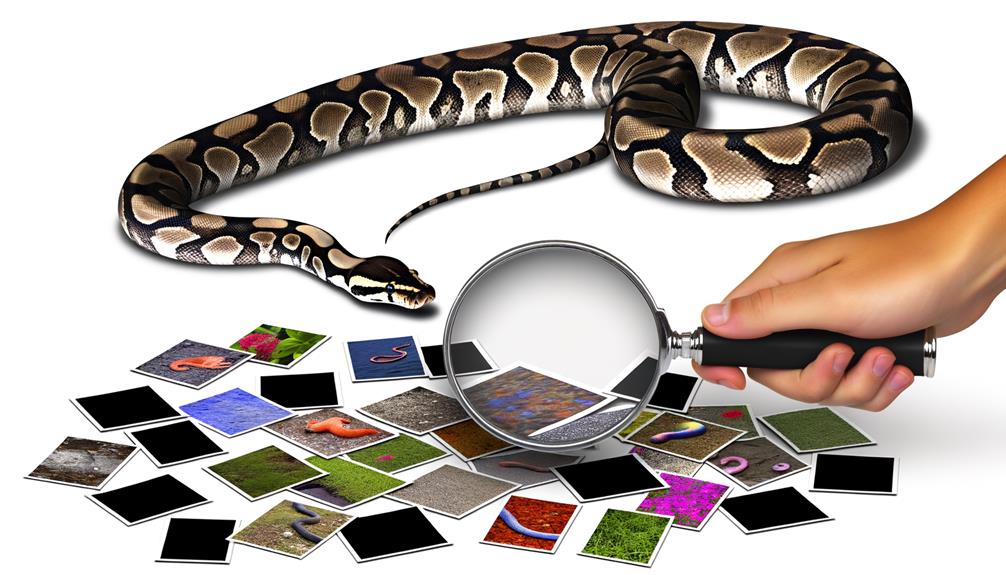
Now that we've installed the necessary Python libraries, let's dive into the process of writing a script that will enable us to resize images in bulk efficiently. Our primary goal is image resizing automation, saving time and reducing manual effort. The script will loop over each image in a specified directory, resize it according to our preset dimensions, and save the output in a new folder. This way, we're not only resizing multiple images at once but also maintaining the original images intact. It's important to note that our script should handle exceptions, like non-image files in the directory, to prevent any runtime errors. This bulk resizing strategy is a powerful tool for managing large image datasets.
Implementing Resizing Parameters
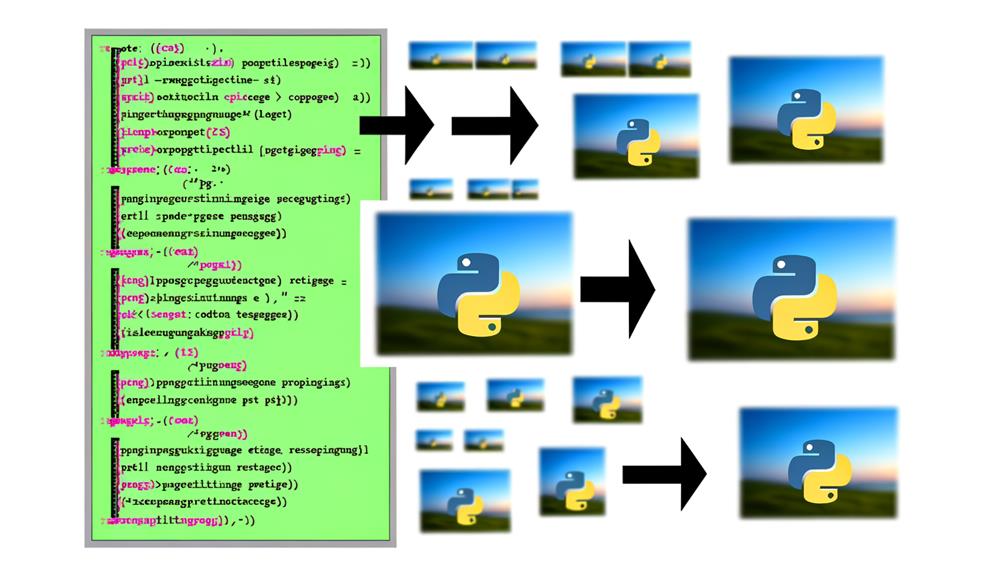
Determining and implementing the right resizing parameters is a crucial step in our bulk image resizing process, as it ensures consistency across all images and allows us to maintain a specific aspect ratio. With dynamic resizing, we're able to adapt these parameters based on the specific needs of each image.
- Dynamic Resizing: This involves establishing a set of rules that adapt to each image's unique dimensions, ensuring that no image is distorted or loses its quality during the resizing process.
- Maintaining Aspect Ratio: By keeping the aspect ratio consistent, we ensure that images do not appear stretched or squashed, preserving their original aesthetic quality.
- Resizing Parameters Implementation: After determining the parameters, we implement them into our Python script, which automates the resizing process, saving us time and effort.
Optimizing Resizing Scripts

To further streamline our image resizing process, we delve into the optimization of our Python scripts, focusing on aspects such as dynamic resizing parameter specification, error handling, and performance optimization. Our dynamic parameterization strategies allow us to adjust resizing parameters flexibly based on the specific image dataset, ensuring optimal results. We employ advanced error handling techniques, including exception handling and input validation, to make our scripts robust against possible errors. Additionally, we're keen on improving the speed and efficiency of our scripts. By profiling our codes, we identify bottlenecks and optimize them through techniques such as loop unrolling and function inlining. In conclusion, the optimization of our Python scripts is a meticulous process that balances flexibility, robustness, and performance.
Parallel Processing for Large Images

How can we tackle the challenge of resizing large sets of images more efficiently? The answer lies in parallel processing, which optimizes CPU usage and assists in handling memory constraints.
- Multiprocessing: By using Python's multiprocessing library, we can divide the task of resizing images across multiple cores, thus reducing the time taken significantly.
- Memory Management: Large images can consume substantial memory. We'll need to keep a close watch on memory usage and release memory resources when not in use to avoid system sluggishness or crashes.
- Asynchronous Processing: We can perform resizing operations asynchronously, allowing us to start other tasks before the current one finishes.
Thus, through parallel processing, we can efficiently resize large image sets, optimizing CPU and memory usage.
Frequently Asked Questions
What Are the Differences Between PIL and Pillow Libraries for Image Resizing?
We've observed that PIL and Pillow both offer robust image manipulation capabilities. However, Pillow is essentially an improved version of PIL, offering added functionality and better performance. It's simpler to install, supports more image formats, and is actively maintained. So, if you're comparing PIL vs Pillow performance, we'd recommend Pillow for its enhanced features and ease of use, especially for tasks like image resizing.
Can Python Libraries Handle Resizing of Vector Images or Only Raster Images?
We've found that Python libraries generally excel at resizing raster images, not vector images. Raster images are like puzzles, each pixel a piece we can manipulate. Vector images, however, are more like fluid blueprints, their shapes defined by mathematics. Library compatibility largely depends on this distinction. So, for vector manipulation, we'd look towards libraries designed for that, such as Matplotlib or Pygame, rather than image-specific ones like Pillow or PIL.
How Does Resizing Affect the Metadata of an Image?
When we resize an image, the metadata often stays intact. But, it's not always guaranteed, especially when using certain libraries or techniques. The impact on image quality can vary, too. Some resizing methods may degrade quality. However, metadata preservation techniques can ensure important information isn't lost. It's crucial to consider this when choosing our image resizing approach.
What Are the Limitations of Pythons Image Resizing Libraries?
We've noticed some limitations with Python's image resizing libraries. They sometimes struggle with efficiency in their resizing algorithms, especially when dealing with large batches of images. Additionally, image quality preservation can be an issue. While resizing, there's often a loss of detail, resulting in less-than-ideal image quality. However, different libraries tackle these issues in varied ways, so choosing the right one for your needs can mitigate these limitations.
Are There Any Python Libraries Specifically Designed for Resizing Images in Specific Formats, Like JPEG or Png?
Are there Python libraries designed for specific image formats? Absolutely! When comparing libraries, Pillow stands out for its JPEG and PNG format compatibility. We've found it's versatile and efficient for resizing these formats. With Pillow, we're not just limited to one format, enhancing our image processing capabilities. So, in terms of library comparisons and format compatibility, Pillow definitely tops our list for JPEG and PNG image resizing.